5 Breaking Changes JavaScript Features in ES15 (2024)
With each new ECMAScript release, JavaScript evolves to offer more powerful and efficient features. The upcoming ECMAScript 2024 (ES15) introduces several groundbreaking changes that will impact the way developers write and structure their code. In this article, we’ll explore five major breaking changes that come with ES15, and why they matter for developers looking to adopt the latest JavaScript standards.
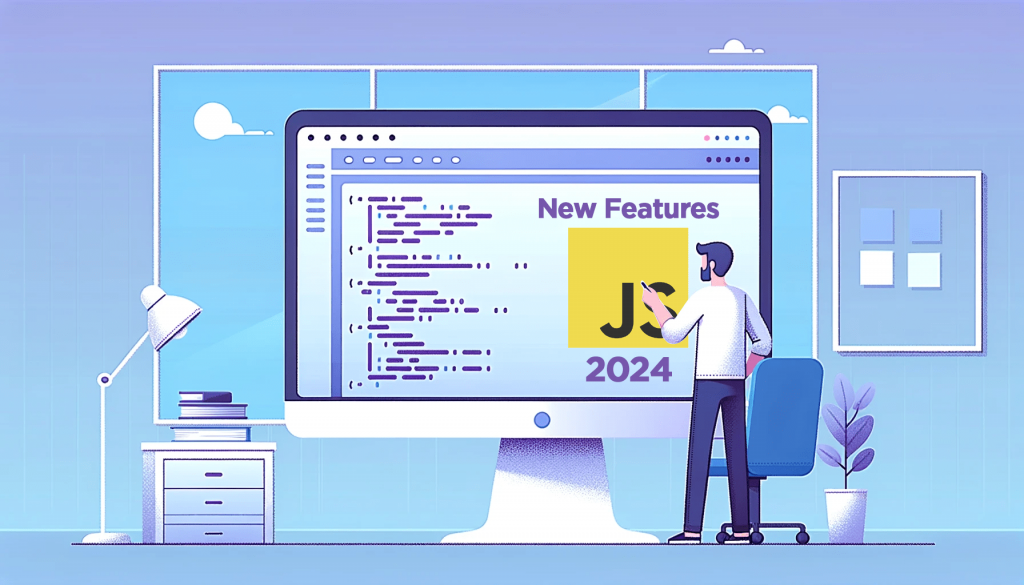
1. Native Array Group-By
One of the most exciting additions in ES15 is the native Array groupBy() method, which allows developers to easily group array elements based on specific criteria.
What is It?
The groupBy() method creates an object where the keys are determined by a callback function, and the values are arrays of elements that correspond to each key.
How to Use It?
const users = [
{ name: 'Alice', role: 'admin' },
{ name: 'Bob', role: 'user' },
{ name: 'Charlie', role: 'admin' }
];
const groupedByRole = users.groupBy(user => user.role);
console.log(groupedByRole);
// Output:
// {
// admin: [{ name: 'Alice', role: 'admin' }, { name: 'Charlie', role: 'admin' }],
// user: [{ name: 'Bob', role: 'user' }]
// }
Why This is Important
Previously, developers would manually write functions to group array elements, often making code verbose and error-prone. The new native method streamlines this process. However, the addition of groupBy() may lead to conflicts in legacy codebases that have implemented custom groupBy() methods, making this a potential breaking change. 😎
I used to install Lodash or write my own functions to handle only group by object data 😫. But now that Javascript has added GroupBy() to native, I do not worry about this. It is awesome 👏
2. Pipeline Operator (|>)
The pipeline operator is a long-anticipated feature that allows for cleaner function chaining by reducing nested function calls.
What is It?
The |> operator allows developers to pass the result of one function directly into another, creating a more readable flow.
How to Use It?
const result = 5
|> double
|> increment
|> square;
console.log(result); // Output depends on functions used
Why This is Important
The pipeline operator removes the complexity of deeply nested function calls, improving readability. However, its introduction may break existing codebases where developers have defined similar custom operators, leading to confusion in their implementation.
3. Async Context Propagation
ES15 introduces a new model for async context propagation, a feature that allows async execution contexts to automatically pass data between async tasks, making managing state across asynchronous code much easier.
What is It?
It provides a standardized way to propagate context (such as request IDs or authentication tokens) across async calls without the need for manual management.
How to Use It?
import { AsyncLocalStorage } from 'async_hooks';
const asyncLocalStorage = new AsyncLocalStorage();
asyncLocalStorage.run({ requestId: 123 }, async () => {
console.log(asyncLocalStorage.getStore().requestId); // Output: 123
});
Why This is Important
Developers no longer have to manually manage passing state across async tasks, making it easier to write cleaner and more maintainable code. However, the new model could conflict with custom solutions or libraries that have tackled async context propagation in earlier JavaScript versions, leading to compatibility issues.
4. Enhanced JSON Modules
With ES15, JSON modules are now supported as first-class citizens in the module system, allowing you to import JSON files directly into your JavaScript without additional tooling.
What is It?
You can now import JSON data directly, just like importing JavaScript modules.
How to Use It?
import config from './config.json' assert { type: 'json' };
console.log(config.database); // Output: based on the JSON content
Why This is Important
In previous ECMAScript versions, developers relied on bundlers or loaders to import JSON files. ES15 makes this part of the standard module system, simplifying configuration-heavy apps. However, this change might break code that relies on older, non-standard ways of importing JSON, or if certain build tools are configured with older behaviors.
5. Method Chaining Operator (?.())
ES15 expands optional chaining by introducing the Method Chaining Operator, which adds safety to method invocation in deeply nested objects.
What is It?
The method chaining operator (?.()) allows you to safely invoke methods on potentially null or undefined objects, avoiding runtime errors.
How to Use It?
const obj = {
user: {
getName: () => 'Alice'
}
};
console.log(obj.user?.getName?.()); // Output: 'Alice'
console.log(obj.user?.getAge?.()); // Output: undefined (instead of throwing an error)
Why This is Important
Before, developers had to rely on cumbersome checks to ensure that deeply nested methods existed before calling them. The method chaining operator eliminates the need for such checks, making code more concise and robust. However, it may introduce conflicts or errors in projects that use non-standard chaining patterns or polyfills.
Conclusion
The five breaking changes in ES15 (2024) bring powerful new features to JavaScript, improving both functionality and developer productivity. From the introduction of native groupBy() to the long-awaited pipeline operator, these changes simplify coding practices, but they also require careful consideration when integrating them into existing codebases. As with any major update, migrating to ES15 will require a solid understanding of these features and their potential impact on legacy systems.
© 2024 HoaiNho — Nick, Software Engineer. All rights reserved.