🚀 React 19 Deep Dive: A Senior Engineer’s Practical Guide to New Hooks
Introduction
React 19 introduces a powerful set of hooks that simplify state management, form handling, asynchronous data management, and UX improvements. However, implementing them effectively requires careful consideration. Here’s a comprehensive analysis based on real-world experience.
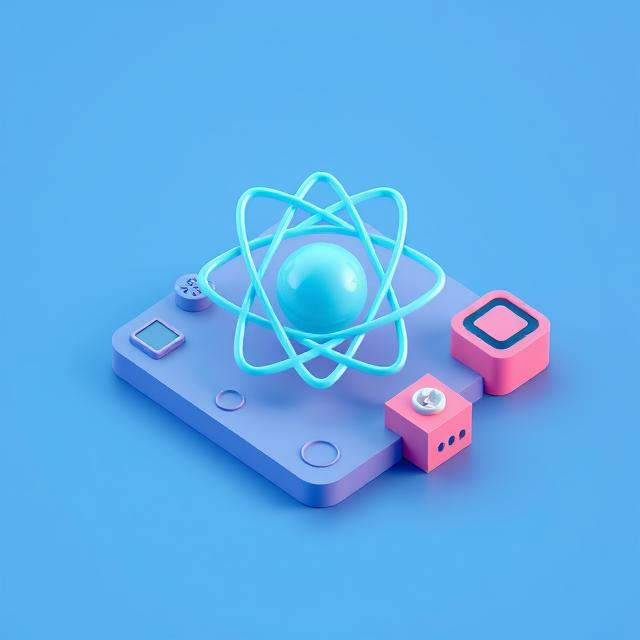
1. The use()
Hook – “A Double-edged Sword in Async Handling”
Real-world Experience
use()
reduces boilerplate but doesn’t completely replaceuseEffect()
+useState()
- Careless implementation can cause “waterfall rendering” (components render slowly due to sequential Promise execution)
- Best utilized in Server Components for optimal performance. Avoid in Client Components with frequently changing data
Practical Implementation
✅ Recommended Use Cases:
- One-time data fetching in Server Components
- Synchronous data reading in Context APIs
❌ Avoid When:
- Handling frequently changing data (e.g., WebSocket data)
- Complex state management beyond
useEffect()
Best Practices:
- ✔️ Wrap components in
<Suspense>
to prevent crash from missing data - ✔️ Ensure proper Promise management to avoid memory leaks
const dataPromise = fetch("/api/user").then(res => res.json());
function UserProfile() {
const user = use(dataPromise); // Single call only
return <div>Welcome, {user.name}!</div>;
}
export default function App() {
return (
<Suspense fallback={<p>Loading user...</p>}>
<UserProfile />
</Suspense>
);
}
2. The useFormState()
Hook – “Good but Not a React Hook Form Replacement”
Real-world Experience
- Makes forms more “stateless” but doesn’t replace powerful form libraries like React Hook Form or Formik
- Works best with server actions. For complex form management, stick with React Hook Form
Practical Implementation
✅ Recommended Use Cases:
- Simple forms with backend validation
- Server-side form handling (excellent for Next.js)
❌ Avoid When:
- Forms requiring complex validation
- Need for focus management, errors, and custom validation
Best Practices:
- ✔️ Combine with
useFormStatus()
for submit state control - ✔️ Always validate server response before UI updates
function MyForm({ action }) {
const [state, formAction] = useFormState(action, { message: "" });
return (
<form action={formAction}>
<input name="email" type="email" required />
<button type="submit">Submit</button>
{state.message && <p>{state.message}</p>}
</form>
);
}
3. The useFormStatus()
Hook – “Small but Mighty”
Real-world Experience
- Extremely useful for checking form submission status without managing separate state
- Limited to
<form>
scope – use Context API for higher-level form tracking
Practical Implementation
✅ Recommended Use Cases:
- Disabling submit buttons during submission
- Displaying loading states
❌ Avoid When:
- Managing multiple form states remotely
Best Practices:
- ✔️ Use only within
<form>
child components - ✔️ Don’t overuse for complex logic – stick to pending state checks
function SubmitButton() {
const { pending } = useFormStatus();
return <button disabled={pending}>{pending ? "Submitting..." : "Submit"}</button>;
}
function MyForm() {
return (
<form>
<input name="email" type="email" required />
<SubmitButton />
</form>
);
}
4. The useOptimistic()
Hook – “Optimistic Engine with Caution”
Real-world Experience
- Excellent for smooth UX but requires careful rollback management
- UI can become out of sync if server returns unexpected data
- Not suitable for critical operations like payments or important data updates
Practical Implementation
✅ Recommended Use Cases:
- Instant UI updates (like buttons, votes)
- Comment/chat lists when sending messages
❌ Avoid When:
- Absolute data accuracy is required (e.g., account balances)
- No rollback mechanism exists
Best Practices:
- ✔️ Always implement rollback for failed requests
- ✔️ Don’t update optimistic state on server errors
function LikeButton({ postId }) {
const [optimisticLikes, setOptimisticLikes] = useOptimistic(0);
async function handleLike() {
setOptimisticLikes(optimisticLikes + 1);
try {
await fetch(`/api/like/${postId}`, { method: "POST" });
} catch (error) {
setOptimisticLikes(optimisticLikes - 1); // Rollback on failure
}
}
return <button onClick={handleLike}>👍 {optimisticLikes}</button>;
}
💡 Summary – “React 19 Changes the Game, but It’s Not a Magic Wand”
When to Use New Hooks?
Hook | When to Use | When to Avoid |
---|---|---|
use() | Server Components data fetching | Client Components, WebSocket |
useFormState() | Simple forms, Server Actions | Complex forms, heavy validation |
useFormStatus() | Submit state checking | High-level form state tracking |
useOptimistic() | Quick UI updates (likes, comments) | Critical data (payments, balances) |
Critical Tips
- ✔️ Use hooks appropriate to context, avoid overuse
- ✔️
use()
is powerful but requires Suspense understanding
Reference Links
- React 19 Documentation
- Server Components Guide
- React Performance Optimization
- Form Handling in React
- React Hooks API Reference
👉 Final Advice: React 19 is a major step forward, but carefully evaluate your real needs before implementation! 🚀
Post Comment
You must be logged in to post a comment.