In Ruby, objects form the core of the language. Everything in Ruby is an object—from numbers and strings to classes themselves. Understanding the Ruby Object Lifecycle can help you write more efficient and effective code, especially when it comes to memory management and performance optimization. In this blog, we’ll explore the different stages of a Ruby object’s lifecycle, from creation to destruction, and offer tips on how to handle these objects efficiently.
What is an Object in Ruby?
In Ruby, an object is an instance of a class, and it encapsulates both data and behavior. Objects can represent anything: numbers, strings, arrays, or even more complex constructs like classes and modules. Each object in Ruby has its unique identity and set of attributes.
Example:
str = "Hello, Ruby!"
puts str.class # Output: String
puts str.object_id # 68300
In this example, "Hello, Ruby!"
is an object of the class String
.
The Stages of a Ruby Object’s Lifecycle
1. Object Creation
The first step in the object lifecycle is creation. When you instantiate a new object, Ruby allocates memory to store that object. This is done through a class’s new
method or by using literal constructors like strings ("string"
), arrays ([]
), or hashes ({}
).
How Object Creation Works:
- Ruby allocates memory for the object on the heap.
- The object is assigned an object ID, which is unique for each object.
- The object’s instance variables are initialized (if any), and default values are set.
Example of Object Creation:
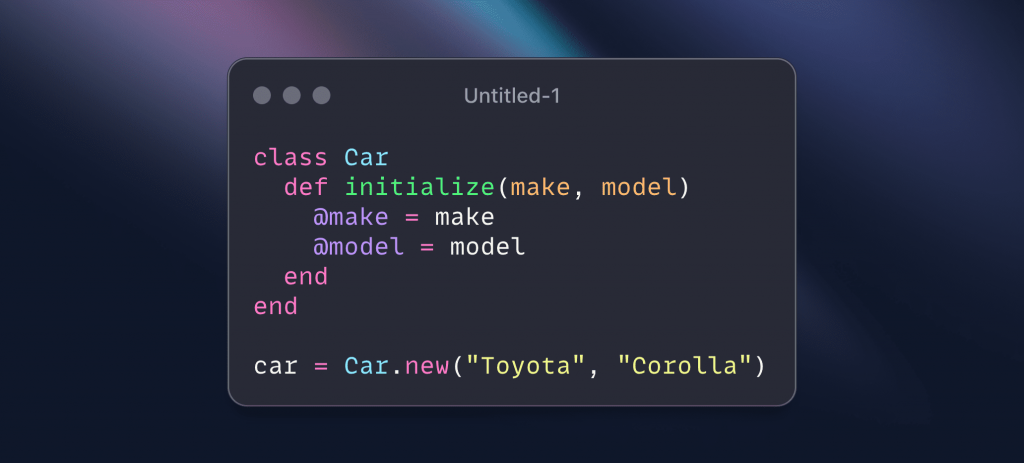
Here, a new Car
object is created with the attributes make
and model
.
2. Object Usage
After creation, the object is now ready for use. This stage is where most of the work happens. You can call methods on the object, change its state, or interact with it in various ways.
- Instance Methods: You can call methods on the object to interact with its data or perform operations.
- State Changes: The state of the object can change by modifying its instance variables.
Example of Object Usage:
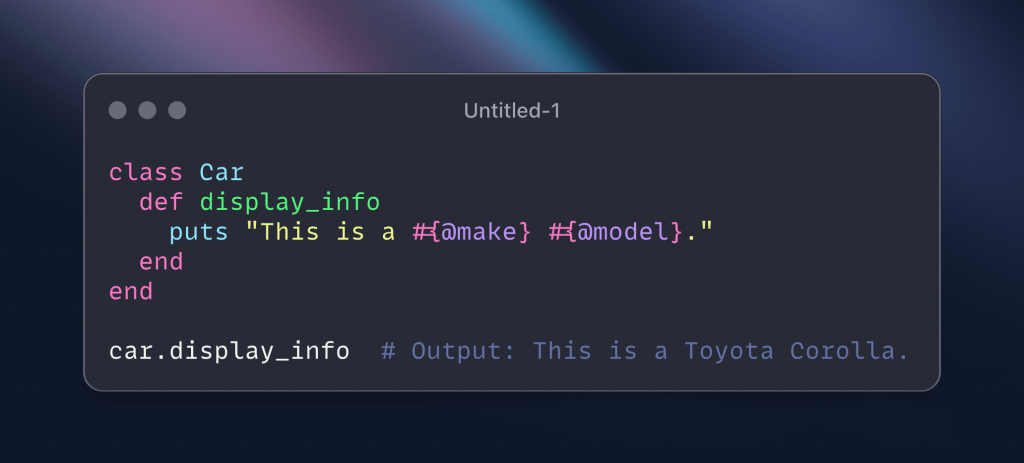
3. Object Destruction (Garbage Collection)
The final stage in an object’s lifecycle is destruction, where the object is no longer needed and its memory is reclaimed by the garbage collector. In Ruby, memory management is handled automatically by the garbage collector (GC), which periodically scans for objects that are no longer referenced by any part of the program.
Key Concepts of Object Destruction:
- Unreferenced Objects: When an object has no remaining references (i.e., no variable or structure is pointing to it), it becomes eligible for garbage collection.
- Garbage Collection: Ruby’s garbage collector frees up the memory associated with unreferenced objects, making it available for new objects.
Example of Object Destruction:
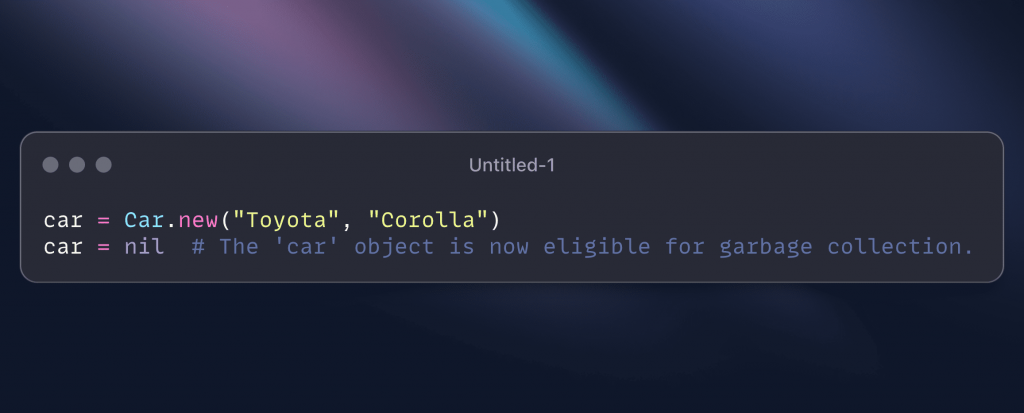
In this example, after setting car
to nil
, the original Car
object is no longer referenced and can be garbage collected.
In this example, the car
object exists only within the create_car
method. After the method finishes executing, the car
variable is no longer accessible, and the object it references becomes eligible for garbage collection.
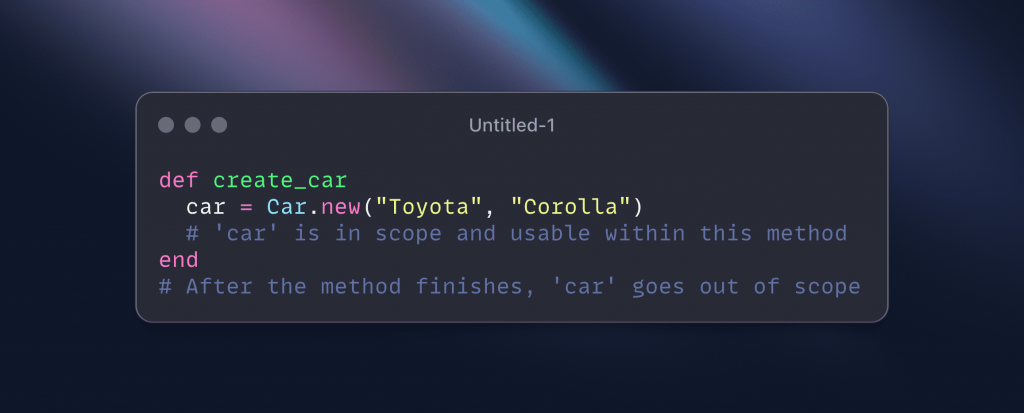
What Happens During Object Destruction (Garbage Collection)?
When an object is no longer referenced:
- Garbage Collection: Ruby’s garbage collector periodically checks for objects that are no longer referenced. When it finds such objects, it reclaims the memory allocated to them.
- Memory Reclamation: The object’s memory is freed, making it available for new objects that may be created later.
- Finalizers (Optional): If a finalizer is defined for an object (using
ObjectSpace.define_finalizer
), it will be called just before the object is destroyed.
Common Misconception: Objects Are Destroyed Immediately
It’s important to note that Ruby does not destroy objects immediately when they go out of scope. The garbage collector works in the background, and destruction happens when the GC decides it’s time to clean up. This means that even if an object is out of scope, it might still linger in memory until the garbage collector runs.
4. Finalizers (Optional)
Although Ruby’s garbage collector takes care of most memory cleanup tasks, you can define a finalizer for objects using the ObjectSpace
module. A finalizer is a callback that gets invoked just before the object is destroyed by the garbage collector. Finalizers are rarely used in practice, but they can be helpful for cleaning up external resources (e.g., closing files or network connections).
Example of a Finalizer:
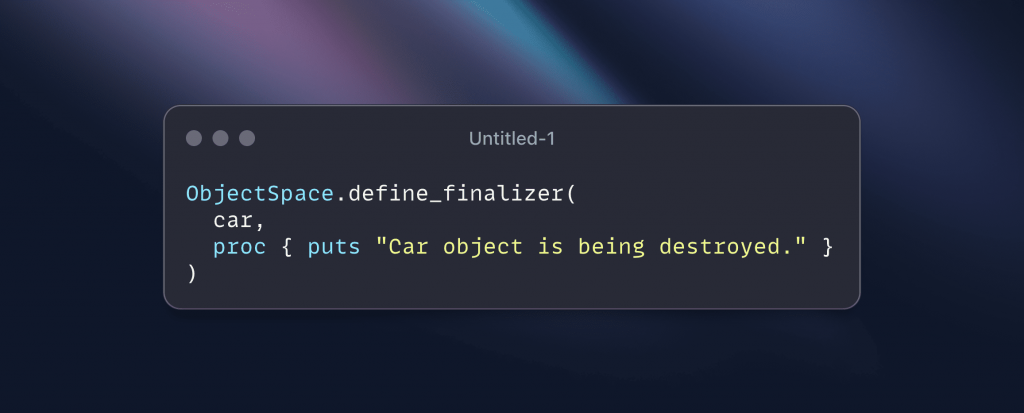
This code will output a message just before the car
object is destroyed.
Understanding Memory Efficiency and Object Lifecycle
Now that you understand the object lifecycle, it’s important to consider how object management affects memory usage in Ruby applications:
- Avoid Excessive Object Creation: Creating too many objects, especially in loops or frequently called methods, can put pressure on the garbage collector and degrade performance.
- Use Object Reuse: Instead of constantly creating new objects, consider reusing existing ones. For example, when dealing with strings, you can use symbols (
:symbol
) instead of string literals ("string"
) to reduce memory consumption, since symbols are immutable and reused by Ruby. - Monitor Garbage Collection: Ruby provides tools like
GC.stat
and theGC::Profiler
to monitor garbage collection events. These tools can help you optimize memory usage by identifying areas where the GC is working too hard.
Example of Using GC.stat
: (https://ruby-doc.org/core-2.7.4/GC.html)
puts GC.stat
This code will display the current garbage collection statistics, allowing you to monitor memory performance.
Object Lifecycle and Performance
Understanding the lifecycle of Ruby objects is crucial for optimizing application performance. When objects are created and destroyed frequently, the garbage collector is triggered more often, which can slow down the application. Conversely, retaining too many objects in memory for long periods can lead to memory bloat.
Tips for Managing Object Lifecycle Efficiently:
- Lazy Initialization: Only create objects when they are actually needed. This avoids unnecessary memory usage.
- Use Caching: Cache objects that are expensive to create or that are reused frequently.
- Profile Memory Usage: Use tools like MemoryProfiler to track memory allocations and detect memory leaks.
Conclusion
In Ruby, understanding the object lifecycle—creation, usage, and destruction—is essential for writing efficient and performant code. While Ruby’s garbage collector handles most of the memory management automatically, knowing how and when objects are created and destroyed can help you avoid memory bloat and optimize application performance.
By reducing unnecessary object creation, reusing objects where possible, and keeping an eye on garbage collection metrics, you can ensure that your Ruby applications run smoothly and efficiently.
Reference: https://ruby-doc.org/core-2.7.4/GC.html