In the world of containerization, building Docker images for different platforms can be essential, especially when your application needs to run on various operating systems and architectures. Docker’s buildx
command extends Docker’s build capabilities by making it easier to build images that support multiple platforms (e.g., Linux, Windows, and ARM).
This blog will guide you through using docker buildx
to build multi-platform Docker images, why it’s beneficial, and how it can improve your DevOps workflow.
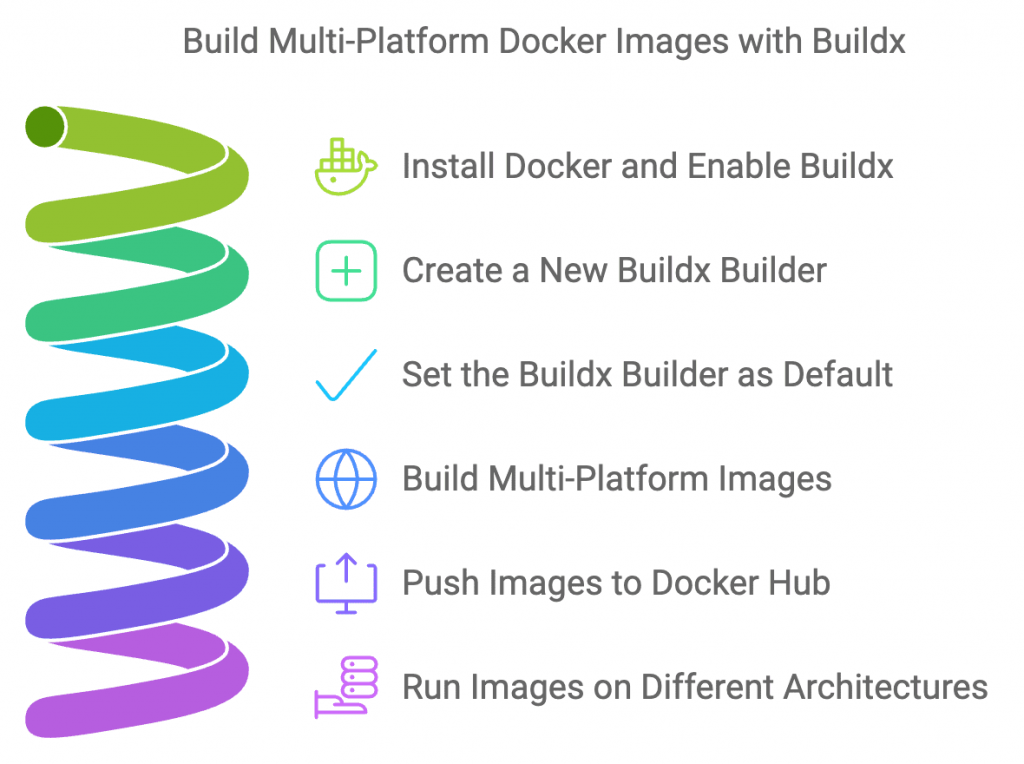
What is Docker Buildx?
Docker buildx
is an experimental feature that enhances Docker’s image-building process. It provides more advanced features like:
- Multi-platform builds: Build an image that can run on different OS platforms and architectures, like
linux/amd64
,linux/arm64
, and more. - Cross-compilation: Build images on a host machine for platforms other than the host’s architecture.
- Cache export/import: Speed up the build process by reusing cached layers from previous builds.
- External Builders: Use containerized builders (like Kubernetes or remote build servers). (read more)
buildx
uses BuildKit, a modern backend for building Docker images that speeds up builds and adds more flexibility to the build process.
Why Use Docker Buildx?
- Multi-platform Compatibility: Create a single image that can be deployed across multiple platforms, avoiding the need to manage different images for each platform.
- Improved Performance: BuildKit’s advanced caching strategies and parallel builds can significantly speed up the image-building process.
- Cross-Architecture Building: You can build images for architectures that are different from your host machine, making it easier to develop for ARM devices like Raspberry Pi.
- Increased Flexibility: Buildx allows the use of external builders, which is useful for CI/CD pipelines or Kubernetes-based environments.
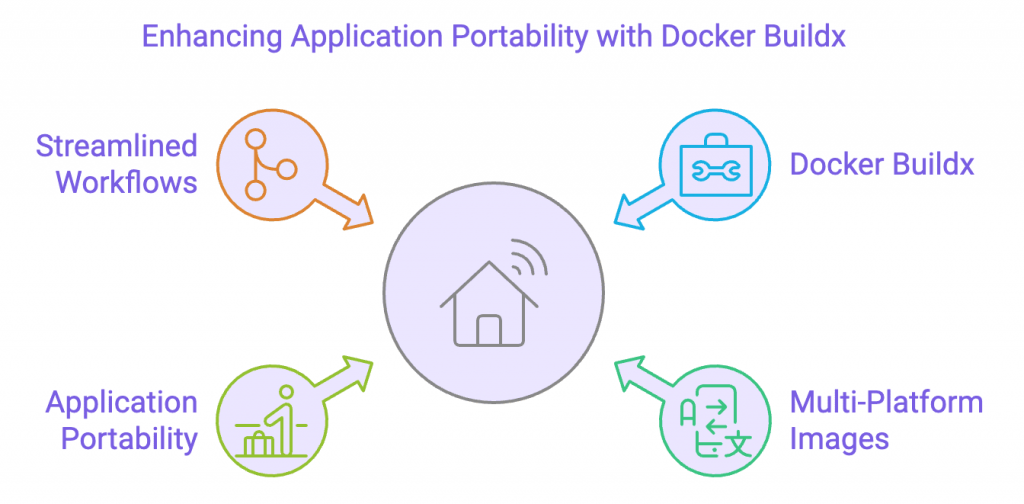
How to Use docker buildx
Step 1: Install Docker Buildx
If you’re using Docker Desktop, Buildx comes pre-installed. However, for Linux or server environments, you might need to install it manually.
To check if buildx
is installed:
docker buildx version
If it’s not installed, you can add it by running:
docker build --install
Step 2: Set Up Buildx Builder
To take full advantage of Buildx, you need to set up a builder instance. This can be a local builder, or you can run a more advanced setup like a remote Kubernetes builder.
To create a new builder instance, run:
docker buildx create --name mybuilder --use
This sets mybuilder
as the active builder.
Step 3: Building a Multi-Platform Image
Once the builder is ready, you can build an image for multiple platforms using the --platform
flag.
docker buildx build --platform linux/amd64,linux/arm64 -t myapp:latest .
In this example, the image will be built for both amd64
(common for x86 machines) and arm64
(popular for ARM processors like Raspberry Pi).
Step 4: Push to a Docker Registry
To push your multi-platform image to a registry (like Docker Hub, GitHub Packages, or your own registry), simply add the --push
flag:
docker buildx build --platform linux/amd64,linux/arm64 -t myregistry/myapp:latest --push .
This will build and push the images for both architectures in a single process.
A Real-World Example
Let’s walk through a practical example where we build a Docker image that supports both linux/amd64
and linux/arm64
platforms and push it to a Docker registry like GitLab.
Here’s the command:
docker buildx build \
--platform linux/amd64 \
--builder kamal-local-docker-container \
-t registry.gitlab.com/sudoxglobal/sudo-auth:6a95a12f8d0e2779fe7cf8b9d430f69e05965cf3 \
-t registry.gitlab.com/sudoxglobal/sudo-auth:latest \
--label service="sudo_auth" \
--file Dockerfile .
In this command:
- We specify
linux/amd64
as the platform for which we are building the image. - We use the
kamal-local-docker-container
as the builder instance. - We tag the image both with a specific commit hash (
6a95a12f8d0e2779fe7cf8b9d430f69e05965cf3
) andlatest
for easy version management. - We label the service as
"sudo_auth"
to ensure it is categorized and identifiable within the registry.
Use Cases for docker buildx
- Cross-Architecture Development: If you’re developing software for ARM-based systems (like Raspberry Pi or mobile devices) but using a traditional x86 machine, you can build images for ARM using
docker buildx
. - Multi-Platform Deployments: If your application needs to run on different platforms (e.g., Windows and Linux),
buildx
allows you to create a single image that works across all platforms. - CI/CD Pipelines: With
docker buildx
, you can simplify your CI/CD pipeline by building and pushing multi-platform images in one go, rather than maintaining separate pipelines for different architectures.
Conclusion
Docker buildx
is a powerful tool for building Docker images that can run on multiple platforms and architectures. It simplifies workflows for developers working in heterogeneous environments and offers enhanced performance and flexibility over traditional Docker builds.
By mastering buildx
, you can optimize your DevOps processes, reduce complexity, and ensure your applications are portable across a variety of devices and platforms.
Compare with docker build
Here’s a comparison table between the traditional docker build
and the advanced docker buildx
:
Feature | docker build | docker buildx |
---|---|---|
Multi-platform support | Limited, requires manual workarounds | Full support for building images for multiple platforms in one command |
Cross-compilation | Not natively supported | Supported, enabling building for different architectures (e.g., ARM on an x86 machine) |
Performance | Slower due to lack of parallel build caching | Faster builds with advanced BuildKit caching and parallelism |
Caching | Basic layer caching | Advanced caching mechanisms (export/import caches for faster builds) |
External builders | Not supported | Supports external builders, allowing builds to run in remote environments like Kubernetes or cloud-based setups |
Push multi-arch images | Not supported natively | Can push multi-architecture images directly to Docker registries with the --push flag |
Multi-node support | No | Yes, allows building on multiple nodes for distributed builds |
Image driver support | Limited to local Docker daemon | Can use different backends (Docker, Kubernetes, containerized builders) |
Custom Build Scripts | Requires additional scripting | Supports advanced features natively, with clean integration of advanced build processes |
Availability | Default in Docker | Requires enabling experimental features and using BuildKit |
Output Formats | Limited to local Docker images | Supports multiple output formats including Docker images, local tar files, or OCI images |