Boosting Backend Performance with Distributed Cache: A Comprehensive Guide
In modern software development, caching plays a vital role in enhancing performance, reducing latency, and ensuring scalability. Among the various caching strategies, distributed cache stands out as a powerful approach for high-traffic applications. This article delves into the fundamentals of distributed cache, compares it with in-memory cache, explains common caching strategies, and includes a practical technical demo with a step-by-step guide for implementation.
What is Distributed Cache?
Distributed cache is a system where cached data is spread across multiple servers or nodes, enabling high availability, fault tolerance, and scalability. Unlike in-memory cache, which stores data on a single node, distributed cache ensures that the caching layer can handle large traffic volumes by distributing the load.
Benefits of Distributed Cache
• Scalability: Add more nodes to handle increasing traffic seamlessly.
• Fault Tolerance: Redundant copies of data ensure availability even during node failures.
• Global Availability: Supports applications deployed across multiple regions.
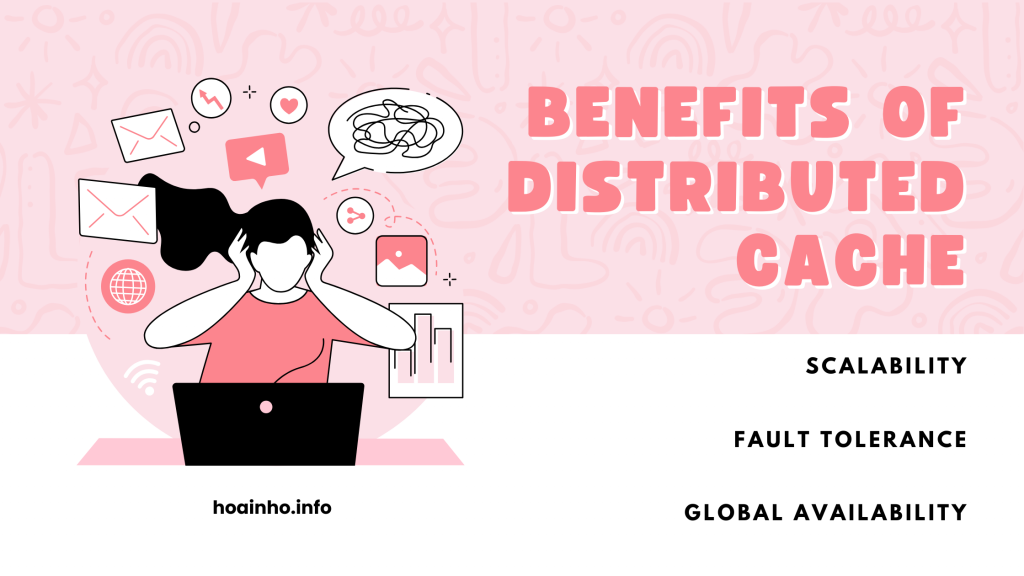
Comparison: Distributed Cache vs. In-Memory Cache
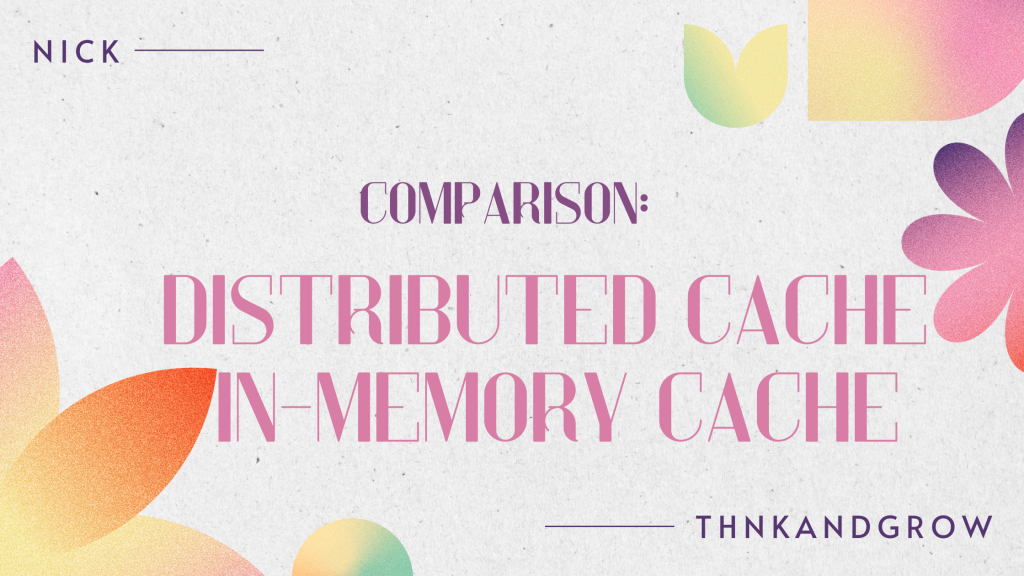
Aspect | In-Memory Cache | Distributed Cache |
---|---|---|
Scalability | Limited to a single machine | Scales horizontally by adding nodes |
Performance | Extremely fast (no network latency) | Slightly slower due to network hops |
Fault Tolerance | None (single point of failure) | High (data replicated across nodes) |
Best For | Small-scale, low-traffic apps | Large-scale, high-traffic systems |
Throughput Comparison:
• In-Memory Cache: Best for up to 10,000 concurrent users.
• Distributed Cache: Designed for over 10,000 concurrent users, excelling under massive traffic.
Common Caching Strategies
Caching strategies define how data is stored, retrieved, and refreshed in the cache. Here are the most popular ones
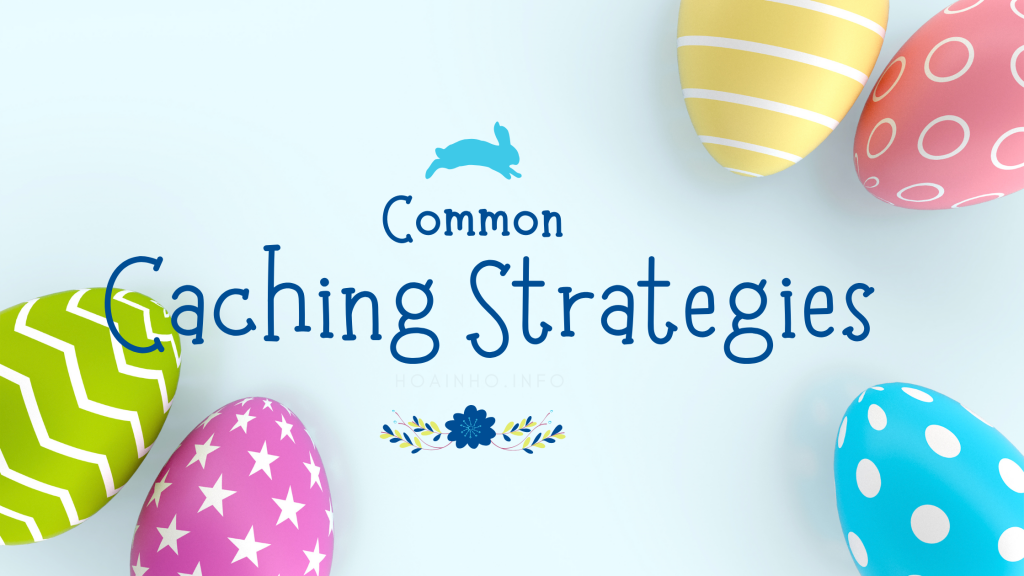
1. Cache-Aside:
Applications check the cache first; if a cache miss occurs, data is fetched from the database and added to the cache.
• Use Case: Dynamic data with frequent updates.
2. Read-Through:
The cache layer handles all reads; it retrieves data from the database if not found in the cache.
• Use Case: Frequently read, rarely updated data.
3. Write-Through:
Data is written to the cache and database simultaneously.
• Use Case: Ensures consistency between cache and database.
4. Write-Behind:
Writes are queued in the cache and asynchronously updated in the database.
• Use Case: High write workloads with acceptable delay in persistence.
5. Time-to-Live (TTL):
Cached data is automatically invalidated after a specified duration.
• Use Case: Temporary data like session tokens or API rate limits.
Technical Demo: Implementing Distributed Cache with Redis
In this demo, we will:
1. Set up a Redis cluster.
2. Practice caching an API endpoint using Redis.
3. Benchmark performance before and after caching.
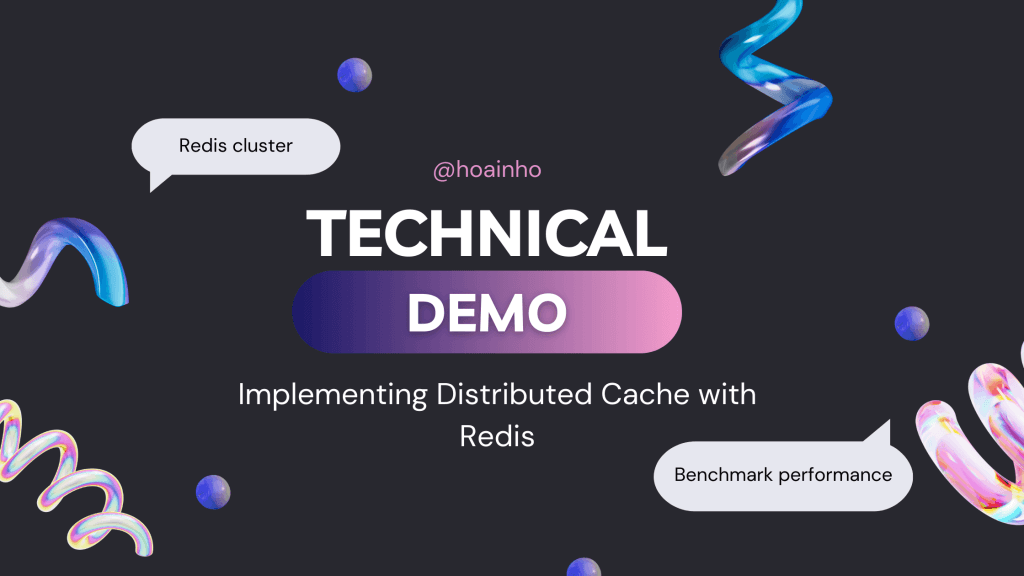
1. Set Up a Redis Cluster
Requirements
• Redis Installation: Install Redis on your local machine or use a managed service like AWS ElastiCache.
• Cluster Configuration: Set up a 3-node Redis cluster (1 master, 2 replicas).
Steps to Set Up
1. Download and Install Redis:
sudo apt update
sudo apt install redis-server
2. Configure Redis for Cluster Mode:
Update the redis.conf file:
cluster-enabled yes
cluster-config-file nodes.conf
cluster-node-timeout 5000
3. Create the Cluster:
redis-cli --cluster create 127.0.0.1:7000 127.0.0.1:7001 127.0.0.1:7002 --cluster-replicas 1
4. Test the Cluster:
Use redis-cli to ensure connectivity:
redis-cli -c -p 7000
Expected Output
A fully functional Redis cluster with master-replica setup.
2. Cache an API Endpoint Using Redis
Scenario
We will cache the response of a product details API (/products/:id) to improve response time.
Implementation (Node.js Example)
1. Install Redis and Dependencies:
npm install ioredis express
2. Code: API with Cache-Aside Pattern
const Redis = require('ioredis');
const express = require('express');
const app = express();
const redis = new Redis();
const getProductFromDB = async (id) => {
// Simulate database query
return { id, name: 'Product ' + id, price: 100 };
};
app.get('/products/:id', async (req, res) => {
const productId = req.params.id;
// Check Redis cache
const cachedProduct = await redis.get(productId);
if (cachedProduct) {
return res.json(JSON.parse(cachedProduct));
}
// Fetch from database
const product = await getProductFromDB(productId);
// Store in cache with TTL
await redis.set(productId, JSON.stringify(product), 'EX', 300);
res.json(product);
});
app.listen(3000, () => console.log('Server running on port 3000'));
3. Benchmark Performance
Testing Tool
Use Apache JMeter or Locust to generate concurrent user traffic.
Metrics to Measure
1. Response Time: Average time for the API to respond.
2. Throughput: Requests handled per second.
3. Database Queries: Number of database hits.
Expected Results
• Without Caching:
• Higher response times (e.g., 100ms).
• Frequent database hits.
• With Caching:
• Lower response times (e.g., 10ms).
• Significant reduction in database queries.
Example Graph
• Plot Response Time vs. Throughput before and after caching.
Conclusion
Distributed caching is a powerful tool for enhancing application performance and scalability. By using strategies like cache-aside and technologies like Redis, you can significantly reduce latency and improve throughput for high-traffic applications. This demo showed how to set up Redis, implement caching for an API, and benchmark the performance gains.
Reference posts:
- 7 Essential Caching Strategies to Boost Backend Performance and Scalability
- Mastering WebSocket Load Balancing: Unlocking the Power of Sticky IPs and Session ID Routing for Seamless Connections
- Mastering Real-Time Scalability: Redis Solutions for Session Management and Load Balancing in High-Traffic Apps
Would you like to explore advanced topics, such as multi-region caching or cache eviction strategies? Let me know in the comments!