Mastering Async Generators in JavaScript: The Ultimate Tool for Streaming Data
Introduction
In modern web applications, handling asynchronous data is a common challenge. Whether you’re working with real-time APIs, paginated data, or streaming large datasets, knowing how to efficiently process asynchronous information is crucial. Enter Async Generators—a powerful, yet often underutilized feature in JavaScript that allows you to handle asynchronous data flows with ease.
In this article, we’ll explore what async generators are, how to use them, and why they’re an essential tool for any JavaScript developer working with streams of data. We’ll also look at a real-world example where async generators can simplify and optimize the code for streaming data from an API.
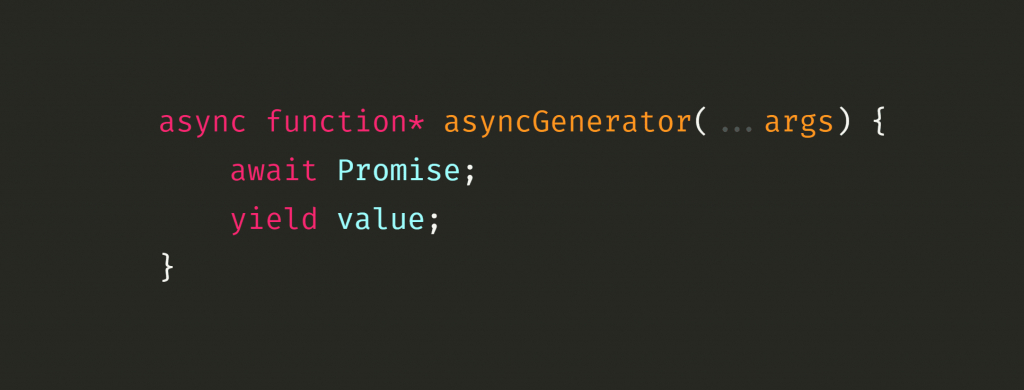
What Are Async Generators?
Async generators are a combination of asynchronous functions and iterators, allowing you to yield values as they become available from asynchronous operations (like network requests or I/O operations). This means you can pause and resume functions while waiting for an asynchronous operation to complete, making it ideal for scenarios involving streams of data.
At its core, an async generator is an async function* that can be iterated over with for await…of, yielding each result asynchronously, as opposed to the typical synchronous for…of loop used for iterating over arrays.
How to Use Async Generators
Let’s break down the steps for using async generators:
- Declare an async generator function using async function*.
- Yield values within the generator as they become available, often after asynchronous calls (e.g., await on a fetch request).
- Iterate over the generator using for await…of, which waits for each asynchronous value before proceeding to the next iteration.
Here’s a basic example of using async generators to fetch data from multiple pages of an API:
async function* fetchPages(urls) {
for (const url of urls) {
const response = await fetch(url); // Asynchronously fetch data
const data = await response.json();
yield data; // Yield the data as it becomes available
}
}
const urls = ["<https://api.example.com/page1>", "<https://api.example.com/page2>"];
(async () => {
for await (const page of fetchPages(urls)) {
console.log(page); // Process each page as it arrives
}
})();
Let me explain above example for guys can have an insights 👆
In this example, each URL is fetched asynchronously, and the result is yielded one at a time. The for await…of loop waits for each page’s data to become available before proceeding to the next one.
Okay I hope above example able to helpful with everyone. Continue we’ll dive deep about reason we needs using Async Generators.
When and Why Should You Use Async Generators?
Async generators shine in scenarios where data arrives in chunks or streams rather than all at once. Here are a few common use cases where async generators excel:
- Streaming Data from APIs: APIs that return paginated results, such as those that list products, users, or posts, can benefit from async generators by processing each page of data as it becomes available.
- Real-Time Data Feeds: In cases where you’re consuming real-time data from web sockets or event streams, async generators allow you to handle each chunk of data in real-time, improving the responsiveness of your application.
- Processing Large Datasets: When working with large datasets that can’t be fully loaded into memory, async generators enable you to process the data incrementally, reducing memory consumption.
- Handling Rate-Limited APIs: If you’re dealing with APIs that enforce rate limits, async generators let you handle requests one at a time, respecting the limits without overloading the API.
Why Choose Async Generators Over Promises?
While promises are great for handling one-time asynchronous operations, async generators are better suited when you need to deal with multiple asynchronous events over time. They provide a cleaner, more structured way to work with sequences of asynchronous actions.
For example, consider an application that fetches multiple pages of data from an API. Without async generators, you’d have to chain promises or use recursion, leading to more complex and harder-to-maintain code.
Real-World Example: Streaming Data from a Paginated API
Let’s imagine we’re building an e-commerce dashboard that needs to display a large list of products. The API provides products in pages of 100 items each. Instead of fetching all the data at once, which could overwhelm the server and lead to memory issues, we can use an async generator to fetch and display each page one at a time.
Here’s how we can implement this using async generators:
// Async generator to fetch each page from the products API
async function* fetchProductPages(baseURL, totalPages) {
for (let page = 1; page <= totalPages; page++) {
const url = `${baseURL}?page=${page}`;
const response = await fetch(url); // Fetch data asynchronously
const data = await response.json();
yield data; // Yield each page's data
}
}
const baseURL = '<https://api.ecommerce.com/products>';
const totalPages = 10; // Assume we know there are 10 pages
(async () => {
for await (const products of fetchProductPages(baseURL, totalPages)) {
console.log('Processing page of products:', products);
// Handle and render each page of products in the UI
renderProducts(products);
}
})();
Explanation:
• The async generator fetchProductPages yields a page of product data every time a new page is fetched from the API.
• The for await…of loop ensures that each page is processed one at a time. This approach avoids overloading the server with multiple requests and prevents consuming too much memory by loading all products at once.
• We can easily render each page of products as they are fetched, improving the user experience by showing data progressively, instead of waiting for all pages to load before displaying anything.
Best Practices for Using Async Generators
- Use in Long-Running Tasks: Async generators are ideal for long-running or ongoing tasks, such as consuming event streams or fetching large paginated datasets.
- Error Handling: Since async generators deal with asynchronous code, ensure proper error handling with try…catch inside the generator function to catch and handle potential errors from asynchronous operations like network requests.
async function* fetchWithErrorHandling(urls) {
for (const url of urls) {
try {
const response = await fetch(url);
if (!response.ok) throw new Error(`Error fetching ${url}`);
const data = await response.json();
yield data;
} catch (error) {
console.error(error);
}
}
}
- Graceful Shutdown: If you’re dealing with streams or real-time data, ensure that your async generator can gracefully shut down when the stream ends or the user navigates away.
Conclusion
Async generators are a hidden gem in JavaScript, offering a powerful tool for handling asynchronous data streams in a clean and structured way. They help you break away from complex promise chains and simplify code that deals with sequential asynchronous actions, like paginated API calls or real-time data streams.
By combining async generators with the flexibility of JavaScript and the type-safety of TypeScript, you can create highly efficient, scalable, and maintainable solutions for modern web applications.
Now that you know the secrets of async generators, it’s time to apply them to your projects. Whether you’re working with streaming data, handling paginated APIs, or managing real-time feeds, async generators can elevate your JavaScript code to the next level. Give it a try and unlock the full potential of asynchronous programming in JavaScript.
© 2024 HoaiNho — Nick, Software Engineer. All rights reserved.