Mastering 7 Types of Loading in Frontend Development: Best Practices and Examples
1. Eager Loading
Eager loading fetches resources or data immediately when the application or page is rendered. It works by downloading resources like images, scripts, or data requests as soon as the browser encounters them during page load. This is critical for above-the-fold content or essential functionality.
Why Use It:
To ensure critical resources are available immediately and to avoid delays in rendering key content.
Best Practices:
• Use eager loading only for critical resources.
• Avoid using it for large or non-essential resources to prevent slowing down the initial page load.
React.js Code Example:
import React from "react";
const EagerLoadedImage = () => {
return (
<img
src="/images/hero.jpg"
alt="Hero"
style={{ width: "100%", height: "auto" }}
/>
);
};
export default EagerLoadedImage;
2. Lazy Loading
Lazy loading delays the loading of resources until they are needed, such as when they are scrolled into view. It works by using browser APIs like IntersectionObserver or native attributes (loading=”lazy”) to load resources only when they become visible.
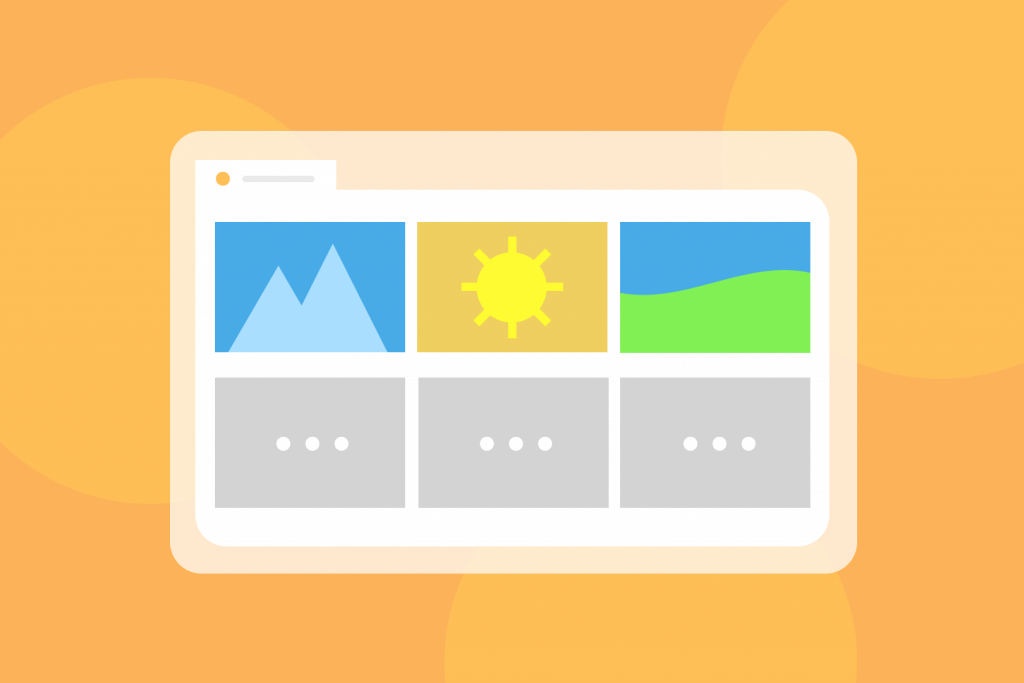
Why Use It:
To improve initial load time and reduce bandwidth usage by loading resources only when necessary.
Best Practices:
• Use lazy loading for images, videos, or components that aren’t visible above the fold.
• Combine with responsive loading techniques for better performance.
React.js Code Example:
import React, { useState, useEffect } from "react";
const LazyLoadedImage = () => {
const [isVisible, setIsVisible] = useState(false);
const imgRef = React.useRef();
useEffect(() => {
const observer = new IntersectionObserver(([entry]) => {
if (entry.isIntersecting) {
setIsVisible(true);
}
});
if (imgRef.current) {
observer.observe(imgRef.current);
}
return () => {
if (imgRef.current) {
observer.unobserve(imgRef.current);
}
};
}, []);
return (
<div ref={imgRef} style={{ minHeight: "300px" }}>
{isVisible && (
<img src="/images/lazy-image.jpg" alt="Lazy" style={{ width: "100%" }} />
)}
</div>
);
};
export default LazyLoadedImage;
3. Code Splitting
Code splitting divides your code into smaller chunks that are loaded on demand rather than in one large bundle. It works using bundlers like Webpack or Vite that automatically split your JavaScript files into smaller bundles.
Why Use It:
To reduce the size of the initial load and improve performance, especially for large applications.
Best Practices:
• Use dynamic imports for routes or components that aren’t needed during the initial load.
• Optimize with React’s React.lazy and Suspense for a seamless user experience.
React.js Code Example:
import React, { Suspense, lazy } from "react";
const LazyComponent = lazy(() => import("./LazyComponent"));
const App = () => {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
};
export default App;
4. Skeleton Loading
Skeleton loading uses a placeholder to mimic the structure of the content being loaded. It works by displaying a skeleton UI that disappears when the actual content is rendered.
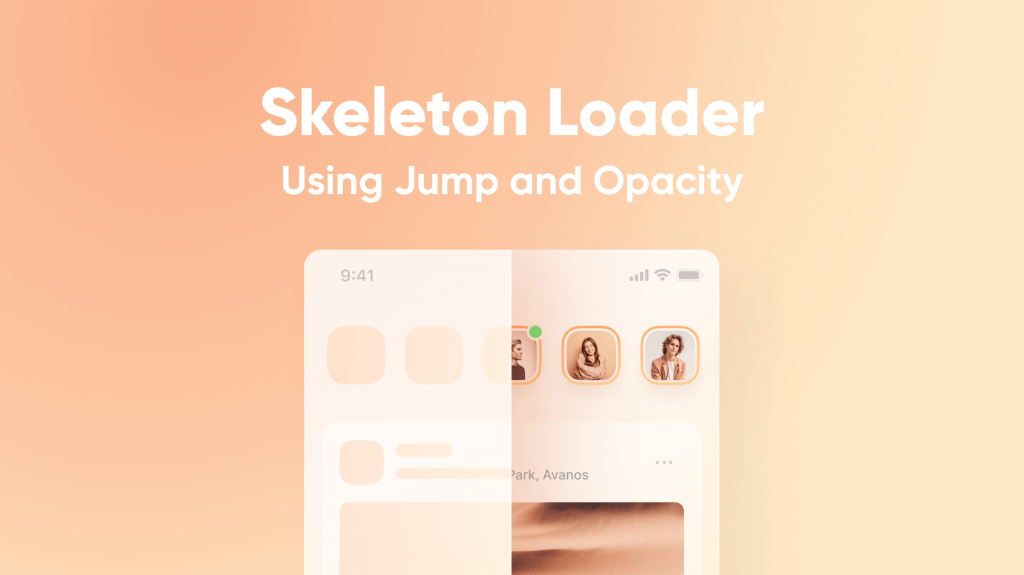
Why Use It:
To improve perceived performance and provide a better user experience during loading states.
Best Practices:
• Use skeleton loading for components or data that take longer to load, such as user profiles or images.
• Keep the skeleton design simple and visually similar to the final content.
React.js Code Example:
import React, { useState, useEffect } from "react";
const SkeletonLoader = () => {
const [isLoading, setIsLoading] = useState(true);
useEffect(() => {
const timer = setTimeout(() => setIsLoading(false), 2000);
return () => clearTimeout(timer);
}, []);
return isLoading ? (
<div className="skeleton" style={{ width: "100%", height: "200px", background: "#ddd" }} />
) : (
<img src="/images/content.jpg" alt="Content" style={{ width: "100%" }} />
);
};
export default SkeletonLoader;
5. Progressive Loading
Progressive loading involves loading resources in stages, such as downloading lower-quality images first and then replacing them with higher-quality versions. It works by using techniques like progressive JPEGs or chunked data transfer.
Why Use It:
To provide users with partial content quickly, improving the perceived loading speed.
Best Practices:
• Use progressive images for media-heavy pages.
• Combine with lazy loading to load higher-quality resources as needed.
React.js Code Example:
import React, { useState } from "react";
const ProgressiveImage = ({ lowResSrc, highResSrc }) => {
const [loaded, setLoaded] = useState(false);
return (
<div>
<img
src={lowResSrc}
alt="Low Res"
style={{ filter: "blur(10px)", position: "absolute" }}
/>
<img
src={highResSrc}
alt="High Res"
onLoad={() => setLoaded(true)}
style={{ opacity: loaded ? 1 : 0, transition: "opacity 0.5s" }}
/>
</div>
);
};
export default ProgressiveImage;
6. Infinite Scrolling
Infinite scrolling loads content continuously as users scroll down the page. It works by dynamically fetching and appending new data to the DOM when the user reaches the bottom of the page.
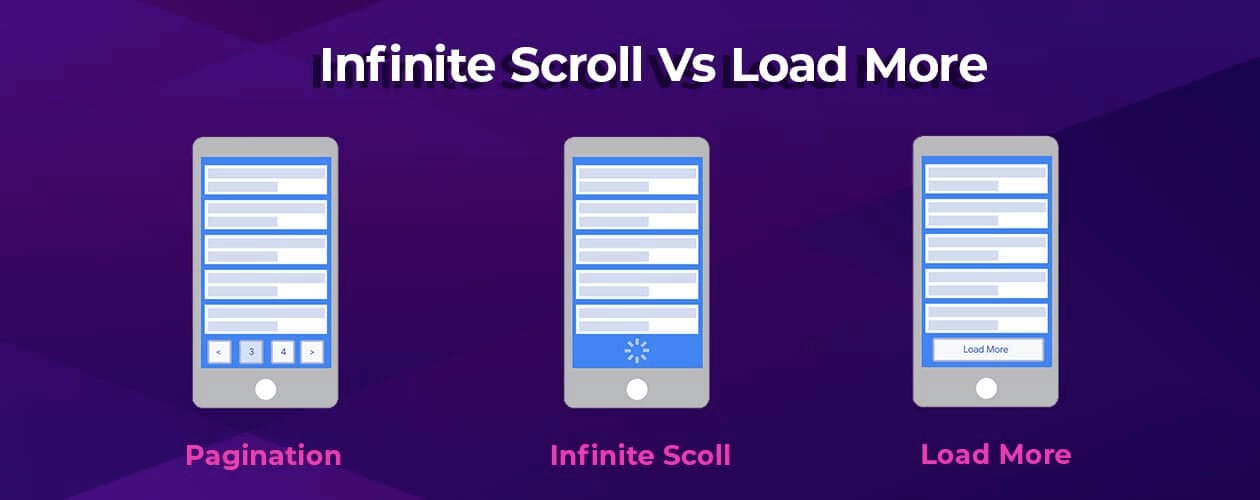
Why Use It:
To improve user experience in applications with large datasets, like social media feeds or e-commerce.
Best Practices:
• Use for content-heavy pages where users expect continuous scrolling.
• Implement proper cleanup to avoid performance issues.
React.js Code Example:
import React, { useState, useEffect } from "react";
const InfiniteScroll = ({ fetchData }) => {
const [data, setData] = useState([]);
useEffect(() => {
const handleScroll = () => {
if (window.innerHeight + window.scrollY >= document.body.offsetHeight) {
fetchData().then(newData => setData(prev => [...prev, ...newData]));
}
};
window.addEventListener("scroll", handleScroll);
return () => window.removeEventListener("scroll", handleScroll);
}, [fetchData]);
return (
<ul>
{data.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
7. Shimmer Loading
Shimmer loading shows an animated gradient placeholder while content is being loaded. It works by displaying shimmering effects to simulate loading activity.
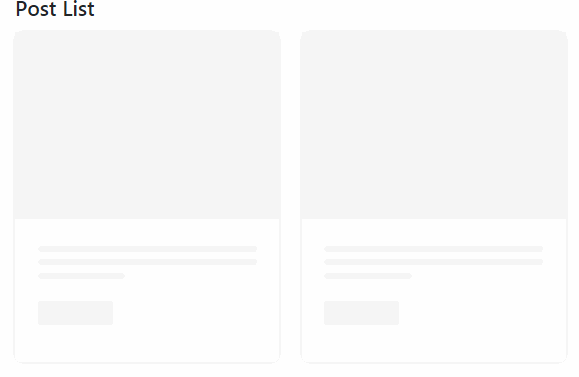
Why Use It:
To create a more dynamic and visually appealing loading experience.
Best Practices:
• Use for components like cards or lists to keep the user engaged.
• Ensure the shimmer animation is lightweight and doesn’t hinder performance.
React.js Code Example:
import React from "react";
const ShimmerLoader = () => {
return (
<div
style={{
width: "100%",
height: "200px",
background: "linear-gradient(90deg, #f0f0f0 25%, #e0e0e0 50%, #f0f0f0 75%)",
backgroundSize: "200% 100%",
animation: "shimmer 1.5s infinite",
}}
/>
);
};
export default ShimmerLoader;