Creating Stylish Folded Tags in ReactJS Using Clip-Path
Custom tags and badges are a great way to add visual flair to your React applications. In this article, we’ll walk through creating a FoldedTag component with unique folded corners using clip-path. We’ll dive into the implementation, styling, and how to use this component effectively.

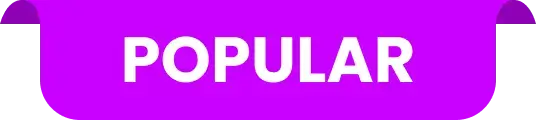
Try to play on Playground
1. Introduction to the FoldedTag Component
The FoldedTag component is a visually appealing badge with folded corners that you can easily customize. It includes:
- Customizable Colors: Adjust the light and dark background colors.
- Optional Folded Corners: Choose whether to display the left or right folded corners.
- Responsive Design: Scalable for different screen sizes.
2. Code for the FoldedTag Component
Component Code (FoldedTag.js)
Here’s the implementation of the FoldedTag component in React:
import React from "react";
import styles from "../styles.module.scss";
import PropTypes from "prop-types";
const FoldedTag = ({ text, color, hiddenFoldLeft = false, hiddenFoldRight = false }) => {
const lightBg = color?.light || "#1c1c22";
const darkBg = color?.dark || "#000000";
return (
<div className="relative inline-flex items-center justify-center">
{/* Left Folded Corner */}
{!hiddenFoldRight && (
<>
<div
style={{ backgroundColor: lightBg }}
className={`absolute top-0 left-0 -translate-x-[9.5px] w-[10px] h-[6px] ${styles.clipFoldedLeft}`}
></div>
<div
style={{ backgroundColor: darkBg }}
className={`absolute top-0 left-0 -translate-x-[9.5px] w-[10px] h-[6px] ${styles.clipPentagonLeft}`}
></div>
</>
)}
{/* Right Folded Corner */}
{!hiddenFoldLeft && (
<>
<div
style={{ backgroundColor: lightBg }}
className={`absolute top-0 right-0 translate-x-[9.5px] w-[10px] h-[6px] ${styles.clipFoldedRight}`}
></div>
<div
style={{ backgroundColor: darkBg }}
className={`absolute top-0 right-0 translate-x-[9.5px] w-[10px] h-[6px] ${styles.clipPentagonRight}`}
></div>
</>
)}
<span
style={{ backgroundColor: lightBg }}
className="text-white uppercase font-bold text-[10px] sm:text-sm rounded-b-[10px] py-1 px-2.5 sm:px-5 whitespace-nowrap"
>
{text}
</span>
</div>
);
};
FoldedTag.propTypes = {
color: PropTypes.object,
text: PropTypes.string,
hiddenFoldLeft: PropTypes.bool,
hiddenFoldRight: PropTypes.bool
};
export default FoldedTag;
3. Styling with SCSS
The folded corners are achieved using clip-path, a powerful CSS property that creates custom shapes. Below is the SCSS file defining the styles for this component:
Styles (styles.module.scss)
.clipPathTriangle {
clip-path: polygon(50% 0%, 100% 100%, 0% 100%);
}
/* Folded left corner */
.clipFoldedLeft {
clip-path: polygon(10% 0%, 10% 0%, 32.592% 2.8%, 51.078% 10.4%, 65.867% 21.6%, 77.363% 35.2%,
85.975% 50%, 92.109% 64.8%, 96.171% 78.4%, 98.57% 89.6%, 99.71% 97.2%, 100% 100%, 100% 0%, 10% 0%);
}
.clipPentagonLeft {
clip-path: polygon(100% 100%, 100% 100%, 99.855% 97.2%, 99.286% 89.6%, 98.091% 78.4%, 96.067% 64.8%,
93.013% 50%, 88.725% 35.2%, 83.002% 21.6%, 75.642% 10.4%, 66.442% 2.8%, 55.2% 0%, 55.2% 0%,
43.667% 2.8%, 33.677% 10.4%, 25.152% 21.6%, 18.014% 35.2%, 12.188% 50%, 7.594% 64.8%, 4.155% 78.4%,
1.795% 89.6%, 0.436% 97.2%, 0% 100%, 100% 100%);
}
/* Folded right corner */
.clipFoldedRight {
clip-path: polygon(90% 0%, 90% 0%, 67.408% 2.8%, 48.922% 10.4%, 34.133% 21.6%, 22.637% 35.2%, 14.025% 50%,
7.891% 64.8%, 3.829% 78.4%, 1.43% 89.6%, 0.29% 97.2%, 0% 100%, 0% 0%, 90% 0%);
}
.clipPentagonRight {
clip-path: polygon(0% 100%, 0% 100%, 0.145% 97.2%, 0.714% 89.6%, 1.909% 78.4%, 3.933% 64.8%, 6.988% 50%,
11.275% 35.2%, 16.998% 21.6%, 24.358% 10.4%, 33.558% 2.8%, 44.8% 0%, 44.8% 0%, 56.333% 2.8%, 66.323%
10.4%, 74.848% 21.6%, 81.986% 35.2%, 87.813% 50%, 92.406% 64.8%, 95.845% 78.4%, 98.205% 89.6%, 99.564%
97.2%, 100% 100%, 0% 100%);
}
4. How It Works
- Clip-Path Shapes: The clip-path property is used to create complex shapes like the folded corners and pentagons.
- Positioning: Absolute positioning is used to place the folded corners in the right locations.
- Customizable Props:
- text: Displays the tag’s text.
- color: Sets the light and dark colors for the tag.
- hiddenFoldLeft and hiddenFoldRight: Enable or disable the folded corners.
5. Usage Example
import React from "react";
import FoldedTag from "./components/FoldedTag";
const App = () => {
return (
<div className="flex flex-col items-center space-y-4">
<FoldedTag
text="New"
color={{ light: "#FF5733", dark: "#C70039" }}
/>
<FoldedTag
text="Sale"
color={{ light: "#33FF57", dark: "#239B56" }}
hiddenFoldLeft
/>
<FoldedTag
text="Hot"
color={{ light: "#3357FF", dark: "#1B4F72" }}
hiddenFoldRight
/>
</div>
);
};
export default App;
6. Conclusion
The FoldedTag component is a stylish way to add custom tags or labels to your React applications. With clip-path, you can create intricate shapes that enhance your UI while keeping the implementation clean and reusable.
Would you like to explore more advanced customization or animations for this component? Let me know through my email: [email protected]
Feel free to customize the colors, shapes, and sizes to suit your needs. Happy coding! 🚀