In Ruby programming, most developers default to using strings for everything from data manipulation to hash keys. However, there’s another option that is often overlooked but can be very helpful in specific situations—Symbols. This blog post will explore the difference between Ruby strings and symbols, and why symbols can sometimes be the better choice. We’ll also provide a performance comparison between symbols and strings.
What Are Symbols in Ruby?
Symbols in Ruby are immutable, lightweight identifiers. They are often used in situations where you would otherwise use a string, especially as hash keys or method names. Unlike strings, symbols are stored in memory only once. This immutability makes symbols more efficient when you need to reference the same label multiple times in a program.
For example, here’s how symbols and strings differ:
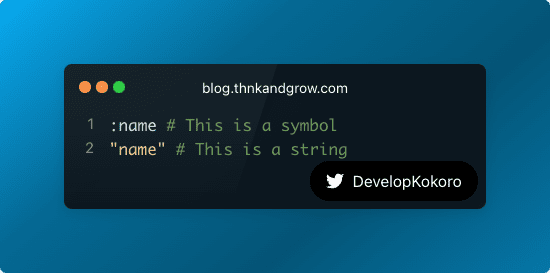
Once a symbol is created, it is stored as a single object for the duration of the program’s runtime. On the other hand, strings are mutable and every time a string is used, it creates a new object in memory (unless explicitly frozen).
Why Symbols Are Rarely Used Instead of Strings
The reason symbols are not used as frequently as strings is that they have a more limited range of use. Strings are flexible—they can be concatenated, modified, and support various string operations. Symbols, on the other hand, are more rigid because they are immutable and cannot be altered after creation.
For example, if you need to modify or manipulate a value, a string is the natural choice:
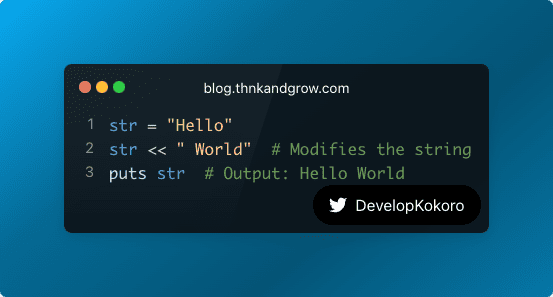
You can’t do this with a symbol. Symbols are more about efficiency than flexibility, which is why they are often used in specific cases where performance or memory usage matters.
When Should You Use Symbols in Ruby?
While strings are more flexible, symbols have some clear advantages when used in certain scenarios. Here are the key areas where symbols are beneficial:
1. Hash Keys
Symbols are commonly used as hash keys because they are stored only once in memory, making them faster for lookups compared to strings. Here’s an example:
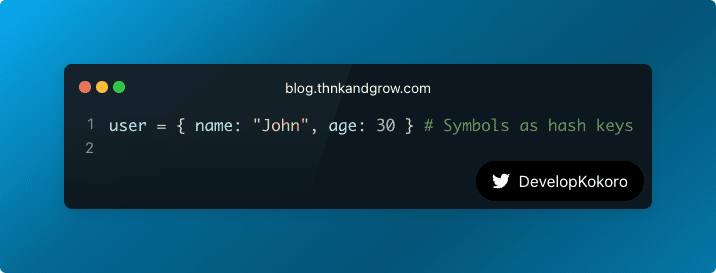
In large datasets or frequently accessed data structures, using symbols as hash keys can improve performance.
2. Method References
Symbols are often used when referencing method names in Ruby, especially in metaprogramming. You might use a symbol to dynamically call a method like this:
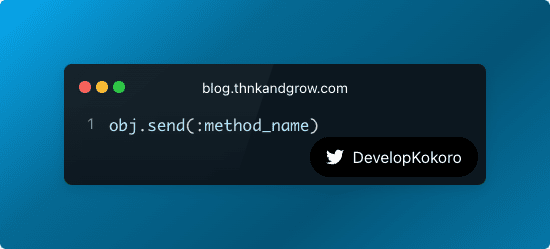
Here, the symbol :method_name
is used to reference the method dynamically. This approach is more memory-efficient compared to using strings.
3. Memory Efficiency
Symbols are immutable and stored only once in memory. When you use a string multiple times in a program, it creates multiple objects, but with symbols, the same object is reused. This can save memory in performance-critical applications.
Performance Comparison: Symbols vs. Strings in Ruby
To understand how much of a difference symbols make in performance, let’s look at a real-world comparison. Below is a benchmark that compares hash lookups using symbols and strings:
Expected Benchmark Results
When you run this code, you’ll likely see that symbol key access is faster than string key access. Here’s a sample output:
String key access time: 0.341772 0.000042 0.341814 ( 0.342158)
Symbol key access time: 0.285133 0.000022 0.285155 ( 0.285392)
This shows that symbols can nearly double the performance when accessing hash keys. The larger the dataset, the more noticeable the performance gains become. Symbols help in reducing memory usage as well, which is important for high-traffic or large-scale applications.
When Not to Use Symbols
While symbols are great for performance, they come with a caveat. Symbols are not garbage collected. If you’re dynamically creating a lot of unique symbols, they will remain in memory for the entire life of the program, which can lead to memory bloat. This is why symbols are best used for static or repeated identifiers, such as method names or configuration keys, and not for dynamic or user-generated data.
If you need to work with dynamic content or perform complex string operations, strings remain the better choice.
Conclusion
In summary, while strings are more commonly used in Ruby due to their flexibility, symbols can offer significant performance improvements when used correctly. Symbols are ideal for use cases such as hash keys, method references, and situations where memory efficiency is important. However, symbols should be used with care—especially in cases involving dynamic data—due to their persistence in memory.
So next time you’re writing Ruby code, think about whether a symbol could be the right choice. When performance matters, using symbols can make a noticeable difference in the efficiency of your application.