JavaScript’s handling of numbers includes limitations due to its use of a 64-bit floating-point format (IEEE 754), which can sometimes lead to unexpected results in large integers and decimal precision. Two useful constants, Number.MAX_SAFE_INTEGER
and Number.EPSILON
, help handle these edge cases effectively. Let’s explore each with examples and use cases.
1. Number.MAX_SAFE_INTEGER
What It Is
Number.MAX_SAFE_INTEGER
represents the maximum integer value that JavaScript can safely handle using its Number
type. Its value is:
Number.MAX_SAFE_INTEGER; // 9007199254740991
This constant equals (2^{53} – 1), which means JavaScript can represent integers safely (without rounding errors) up to this value. Beyond MAX_SAFE_INTEGER
, you may encounter issues with accuracy due to how floating-point numbers are stored.
Example
Consider an example where you’re working with very large integers:
let largeInt = Number.MAX_SAFE_INTEGER; // 9007199254740991
console.log(largeInt); // 9007199254740991
// Adding 1 still works
largeInt += 1;
console.log(largeInt); // 9007199254740992
// Adding 2 starts to show inaccuracies
largeInt += 2;
console.log(largeInt); // 9007199254740994 (Expected: 9007199254740993)
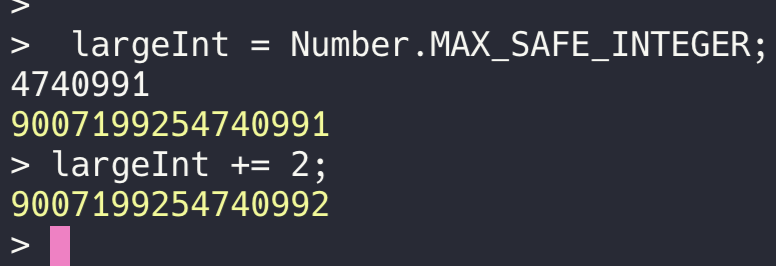
As shown, beyond MAX_SAFE_INTEGER
, JavaScript can no longer accurately represent integers, and adding values starts to produce rounding errors.
Use Cases for Number.MAX_SAFE_INTEGER
- Validation for Large Numbers: When working with APIs or data sources that might return large integers, it’s important to validate them within
MAX_SAFE_INTEGER
if usingNumber
. This ensures they don’t exceed the safe range. - Financial and Precision-Critical Calculations: In applications that handle large integer calculations (e.g., financial systems), use
MAX_SAFE_INTEGER
as a threshold to determine when you might need alternative methods, such asBigInt
or a custom library for arbitrary precision.
Practical Example with Validation
Let’s say you’re processing user input for a large integer ID:
function processId(id) {
if (id > Number.MAX_SAFE_INTEGER) {
throw new Error("The ID exceeds the maximum safe integer range.");
}
// Process the ID safely here
}
If the ID is greater than 9007199254740991
, an error is thrown to prevent inaccuracies.
2. Number.EPSILON
What It Is
Number.EPSILON
represents the smallest difference between 1 and the next possible representable number larger than 1. This value is:
Number.EPSILON; // 2.220446049250313e-16
In JavaScript, Number.EPSILON
is very useful for comparing floating-point numbers because it helps address the tiny errors that can arise from floating-point arithmetic.
Example
Here’s a common example of a floating-point inaccuracy in JavaScript:
console.log(0.1 + 0.2 === 0.3); // false
This is because 0.1 + 0.2
results in 0.30000000000000004
, not 0.3
, due to floating-point precision issues. By using Number.EPSILON
, we can set a tolerance level for comparisons:
function isApproximatelyEqual(a, b) {
return Math.abs(a - b) < Number.EPSILON;
}
console.log(isApproximatelyEqual(0.1 + 0.2, 0.3)); // true
Using Number.EPSILON
, this function checks if two numbers are approximately equal within a tiny range.
Use Cases for Number.EPSILON
- Floating-Point Comparisons: In applications requiring precise decimal comparisons (e.g., scientific calculations),
Number.EPSILON
provides a reliable tolerance. - Avoiding Floating-Point Errors in Financial Apps: For currency calculations or any financial app,
Number.EPSILON
can be used to compare floating-point results, reducing unexpected rounding errors in sensitive calculations.
Practical Example with Precision Comparison
If you have calculations that must be accurate to the smallest possible difference, Number.EPSILON
helps with comparing results:
function checkDiscount(total, expectedDiscount) {
let discount = total * 0.1; // Assume 10% discount
return Math.abs(discount - expectedDiscount) < Number.EPSILON;
}
console.log(checkDiscount(500.0, 50.0)); // true
By using Number.EPSILON
, this function accurately compares discount
and expectedDiscount
, even with floating-point errors.
Summary
Number.MAX_SAFE_INTEGER
is a limit for reliable integer handling. Use it when working with large integer values to avoid rounding errors.Number.EPSILON
helps in comparing floating-point numbers by providing a tolerance range to mitigate tiny arithmetic errors.
Both MAX_SAFE_INTEGER
and EPSILON
are useful constants in JavaScript that help manage numerical limits and precision, making code more robust in handling large integers and accurate floating-point comparisons.