Understanding File Watchers in Rails: Evented vs. Polling-based
In Rails development, efficiently detecting file changes is essential for reloading code and keeping the development process smooth. Rails offers two distinct ways to handle file-watching: ActiveSupport::EventedFileUpdateChecker
and ActiveSupport::FileUpdateChecker
. While both serve the same purpose—tracking changes in the file system—their mechanisms are different, each with its own advantages and trade-offs.
In-depth Comparison
1. ActiveSupport::EventedFileUpdateChecker
:
This is an event-driven file watcher, and it relies on system-level notifications to detect changes in the file system.
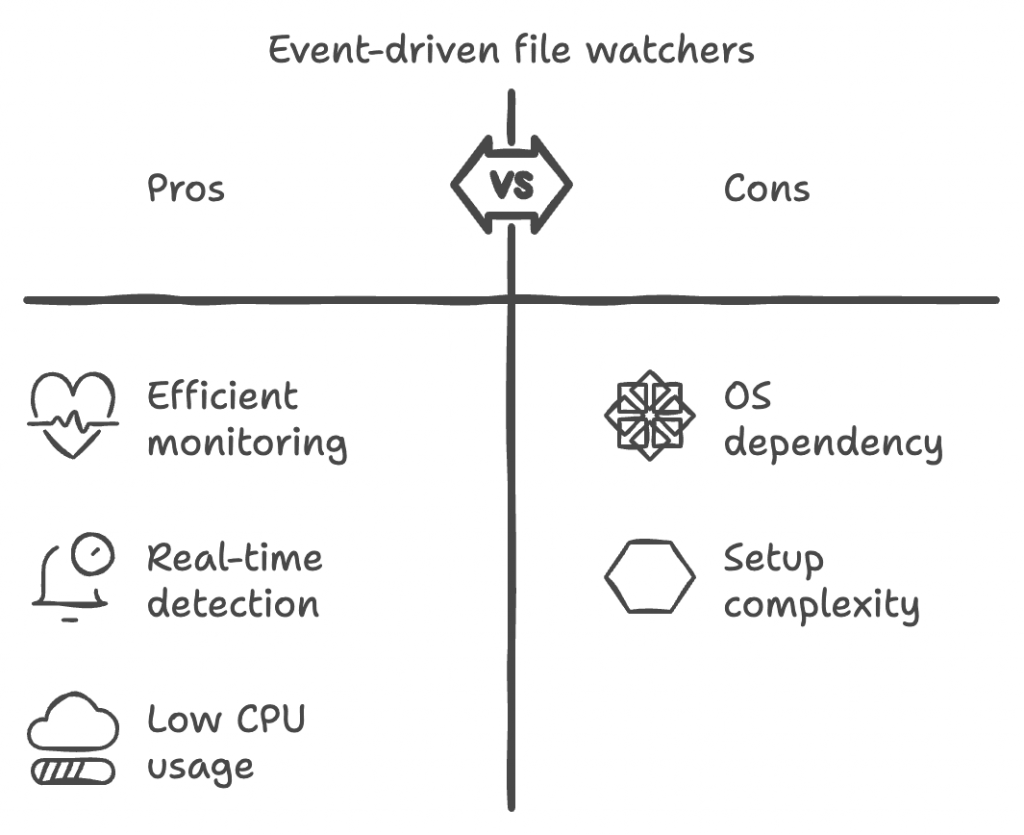
How it Works:
- Event-driven Architecture: It uses the
listen
gem, which is built on top of libraries likerb-inotify
(Linux),rb-fsevent
(macOS), orrb-kqueue
(BSD), depending on the OS. These libraries interact with the file system to listen for changes (like creation, modification, or deletion of files). - Efficient Monitoring: It consumes fewer resources because it waits for the OS to notify it of file changes, rather than manually scanning the file system.
- Real-time Detection: The response to file changes is instantaneous since the system notifies the watcher directly. This makes it ideal for development environments where you want immediate feedback on changes.
- Low CPU Usage: Since it doesn’t have to repeatedly poll for changes, it uses minimal CPU resources, especially when idle.
Pros:
- Efficient resource use: Minimal CPU and I/O overhead because it leverages the OS’s built-in file-watching mechanisms.
- Real-time: Responds immediately to changes.
- Best for Development: It allows for fast, efficient updates while you’re actively developing.
Cons:
- Dependency on external gems: Requires the
listen
gem and platform-specific gems (rb-inotify
,rb-fsevent
, etc.), which may need installation and configuration. - Limited OS support: Some environments, particularly non-desktop or older systems, might not fully support evented file systems.
2. ActiveSupport::FileUpdateChecker
:
This is a simpler, polling-based file watcher.
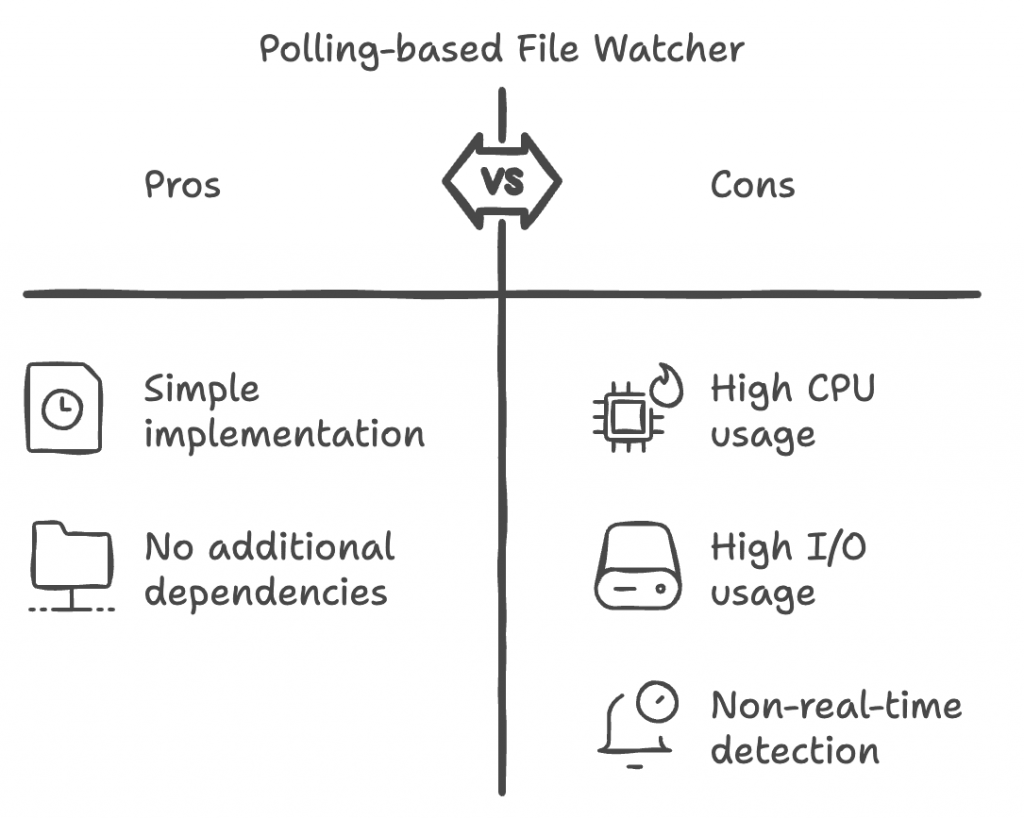
How it Works:
- Polling-based Architecture: It periodically checks the file modification timestamps to detect changes. If a file’s last modified timestamp has changed, it assumes the file has been updated.
- Higher CPU and I/O Usage: Since it constantly checks for changes by polling the file system, it uses more CPU and I/O resources compared to an event-driven system.
- Non-real-time Detection: There is a slight delay in detecting file changes because it only checks for changes at intervals, not instantly upon modification. The frequency of polling can depend on the system’s configuration.
Pros:
- No additional dependencies: Doesn’t rely on external gems like
listen
or platform-specific tools, which makes it more portable across different environments. - Works everywhere: It works on all systems, even in environments where file system notifications are not available (e.g., remote file systems, certain containerized environments).
Cons:
- Less efficient: Due to polling, it consumes more CPU and I/O resources compared to an evented system.
- Slower response: Changes aren’t detected immediately; there’s typically a slight delay.
- Not suitable for large projects: Polling can become expensive in larger applications with many files, as it has to scan through all monitored files regularly.
Detailed Comparison Table:
Feature | ActiveSupport::EventedFileUpdateChecker | ActiveSupport::FileUpdateChecker |
---|---|---|
Mechanism | Event-driven, based on system file system notifications | Polling-based, periodically checks file modification timestamps |
Performance | Very efficient (low CPU and I/O usage) | Less efficient (higher CPU and I/O usage due to regular polling) |
Real-time Detection | Immediate, real-time response to file changes | Slight delay due to polling intervals |
Resource Usage | Minimal resource consumption when idle | Higher resource consumption due to constant polling |
Dependencies | Requires the listen gem and OS-specific libraries like rb-inotify , etc. | No external dependencies, works out-of-the-box |
OS Support | Limited to environments with file system event notification support | Works on all systems, including remote file systems or older environments |
Best Use Case | Development environments, especially for real-time feedback during coding | Environments without event notification support or where polling is acceptable |
Drawbacks | Dependency on external gems, may require installation and configuration | Higher CPU usage, slower change detection |
Use Cases:
ActiveSupport::EventedFileUpdateChecker
:- Development environments: Ideal for Rails development, especially when working locally or in environments that support file system notifications.
- Immediate feedback: Perfect for quick iterations, where changes in code should be detected and reflected instantly without using too many resources.
- Efficient for large projects: As the number of files grows, it remains efficient since it’s event-driven rather than scanning all files.
ActiveSupport::FileUpdateChecker
:- Production environments: While production typically doesn’t use file watchers (because of code reloading concerns), if needed, polling might be more stable for certain platforms.
- Environments without native file-watching support: If you’re working on platforms or environments that don’t support event-based systems (e.g., network-mounted file systems or certain cloud systems), polling might be your only option.
- Small projects: For small apps or projects with few files, polling’s performance overhead is less noticeable.
Recommendation:
For development, ActiveSupport::EventedFileUpdateChecker
is generally recommended due to its efficiency and real-time change detection. However, if you’re working in an environment that doesn’t support event-driven file watching, or if you’re running into issues with listen
or other dependencies, ActiveSupport::FileUpdateChecker
can serve as a reliable fallback.